It remains to be decided whether or not we include a StickMan.
En grund til at inkludere Stickman er at vi bare har MFprinter som eksempel på composition af objekter; Line og GlobalPosition er Value-objekter.
In the this chapter, we show how to define composite objects. For objects this is done by means of part-objects – objects that are fixed parts of the composite object. A part-object is be described using the keyword obj
. For value objects this done using var
or val
. In the following examples, we show how to represent a whole/part-hierarchy using part-objects. +++ evt composition-hierarchy, men det kræver en nærlæsning/omformulering i teksten længere nede hvor vi snakker om whole and parts. Vi får sikkert brug for disse ord.
A first very simple example of object composition is a model of a multi-function printer, that is a printer that in addition to printing also can scan and copy. It consists of a Printer
part-object and a Scanner
part-object.
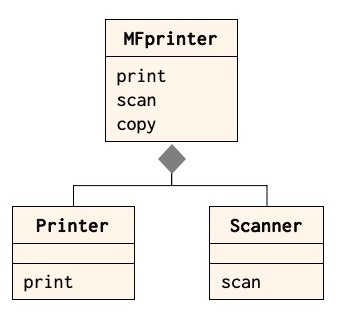
class Printer:
print(doc: ref Document):
...
class Scanner:
scan -> doc: ref Document:
...
class MultiFunctionPrinter:
thePrinter: obj Printer
theScanner: obj Scanner
print(doc: var Document):
thePrinter.print(doc)
scan -> doc: ref Document:
doc := theScanner.scan
copy:
thePrinter.print(theScanner.scan)
The copy
method of the composite MutiFunctionPrinter
uses the properties of the two parts, and it is usually a function that is used directly by a human user. The method print
is invoked by computers linked to a multi-function printer. The scan
-method is typically used by a human who places a document in the scanner and activate the scanner, which sends the scanned document to an email address.
Representing a position on earth
A position of earth is defined using latitude and longitude which both are coordinates from -90 to 90 or shown as 0-90 followed by North/South and East/West. These coordinates may be further spilt into minutes and seconds – often abbreviated DMS standing for degrees, minus and seconds.
We may represent these values using composition of value objects as follows:
GlobalPosition: Value
la: var Latitude
lo: var Longitude
A latitude and longitude may be represented by :
Latitude: Value
theDms: var DMS
theDirection: var NorthSouth
Longitude: Value
theDms: var DMS
direction: var EastWest
And finally a DMS
may be represented by:
DMS: Value
degree: var float
min: var Time.Minutes
sec: var Time.Seconds
The figure illustrates the whole/part hierarchy of a GlobalPosition
-object:
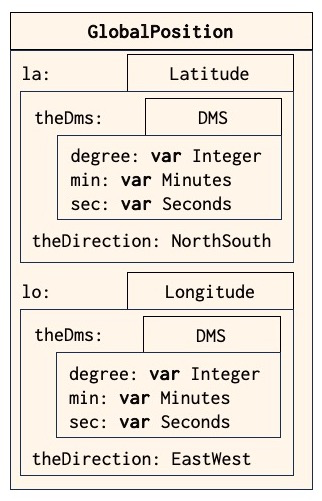
Note that min
and sec
are defined using units of dimension Time
as described in section . The variable degree
has no unit. We leave the definition of NorthSouth
and EastWest
to the reader.
A model of a university
Xiaoping Jia: OO software development using Java has an example with
Student is part of a department, faculty member is part of department, department is part of college, college is part of university.
They say that student and faculty exists independent of the university whereas this is not the case for department and college.
The next example shows part of a representation of a university with the purpose of representing the educations given by the university. A university consists of a number of faculties. Each faculty consist of a number of departments. In this model, a university offers the degrees bachelor, masters and PhD. This structure may be represented in the following way:
class University:
class Faculty:
...
class Department:
...
class Degree:
...
class Bachelor: Degree
...
class Masters: Degree
...
class PhD: Degree
...
arts: obj Faculty
...
naturalScience: obj Faculty
computerScience: obj Department
bachelorInCS: obj Bachelor
... properties special for bachelors in CS ? Find et eksempel
mastersInCS: obj Masters
...
...
physics: obj Department
bachelorInPhysics: obj Bachelor
...
...
...
Note that the faculties Arts, and Natural Science, etc. are represented by singular objects being subclassed from Faculty
. Each faculty has departments belonging to the faculty. Within each department is an objects representing the degrees (bachelor, masters, PhD) offered by the department.
The above example is quite sketchy and we leave to the reader to fill it out to a complete example.
Representing composition
In section , we discussed classification as a fundamental means for organizing and understanding phenomena and concepts. Composition is another fundamental means for organizing and understanding phenomena. Composition is a means to organize phenomena in terms of other phenomena.
In many situations it is useful to consider a whole as constructed from parts. The parts may again consist of smaller, simpler parts i.e. there is a distinction between whole phenomena and their component phenomena. The notion of a whole/part composition is an important means of understanding and organizing complex phenomena.
The part-of relation gives rise to a whole/part hierarchy. Figure xxx above shows an example of such a hierarchy for the stick figure consisting of parts like head, body, arms and legs. In turn, the legs consist of lower leg, foot, etc. vi kan jo innføre alm stickman
A car may be considered as consisting of parts like steering wheel, motor, body and wheels, i.e. wheel is a part of a car, a motor is a part of a car, etc. These components are physical parts of the car. We may represent a car as shown below.
class Car:
aSteeringWheel: obj SteeringWheel
aMotor: obj Motor
aBody: obj Body
wheels: obj Array(4, #Wheel)
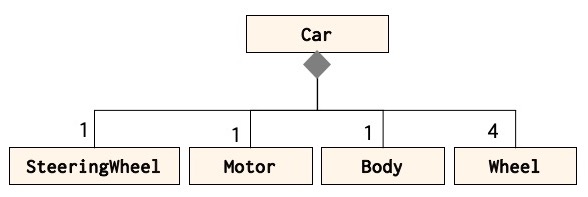
There are numerous other examples of whole/part hierarchies:
- A tree may be viewed as consisting of branches, a trunk, roots and leaves.
- A Hotel Reservation may be viewed as a composition of a Person, a Hotel, a Room and a Date. It is, however, not meaningful to view, for instance, the person as a physical part of the hotel reservation. The corresponding attribute is better viewed as a reference to a person.
- A Person may naturally be viewed as consisting of parts like Head, Body, Arms and Legs. In turn, the Legs consist of Lower leg, Foot, etc.
We define composition in the following way:
Definition xxx To compose is to form a compound phenomenon by means of a number of component phenomena. Properties in the intension of the compound phenomenon are described using the component phenomena. The extension of the compound phenomenon consists of phenomena which have components belonging to the extension of the component phenomena.
To decompose is to identify a component phenomenon of a phenomenon. Decomposition is the inverse of composition.
Composition gives rise to the following relations:
Definition xxx The component-of relation is a relationship between a phenomenon and one of its component phenomena.
Definition xxx The part-of relation is a relation between a phenomenon and one of its part phenomena.
Composition of activities
It is not just physical phenomena as shown in the above examples that may be organised in terms of wholes and parts. This is also the case for activities. An activity described by a method may consist of other activities.
A activity of making a pizza may be understood as a composition of several sub-activities, including: making the dough, making the tomato sauce, preparing the topping, etc.
From the bank domain: Transfer consisting of withdraw and deposit